How To Implement and Use Stacks and Queues in Ruby: The Basics
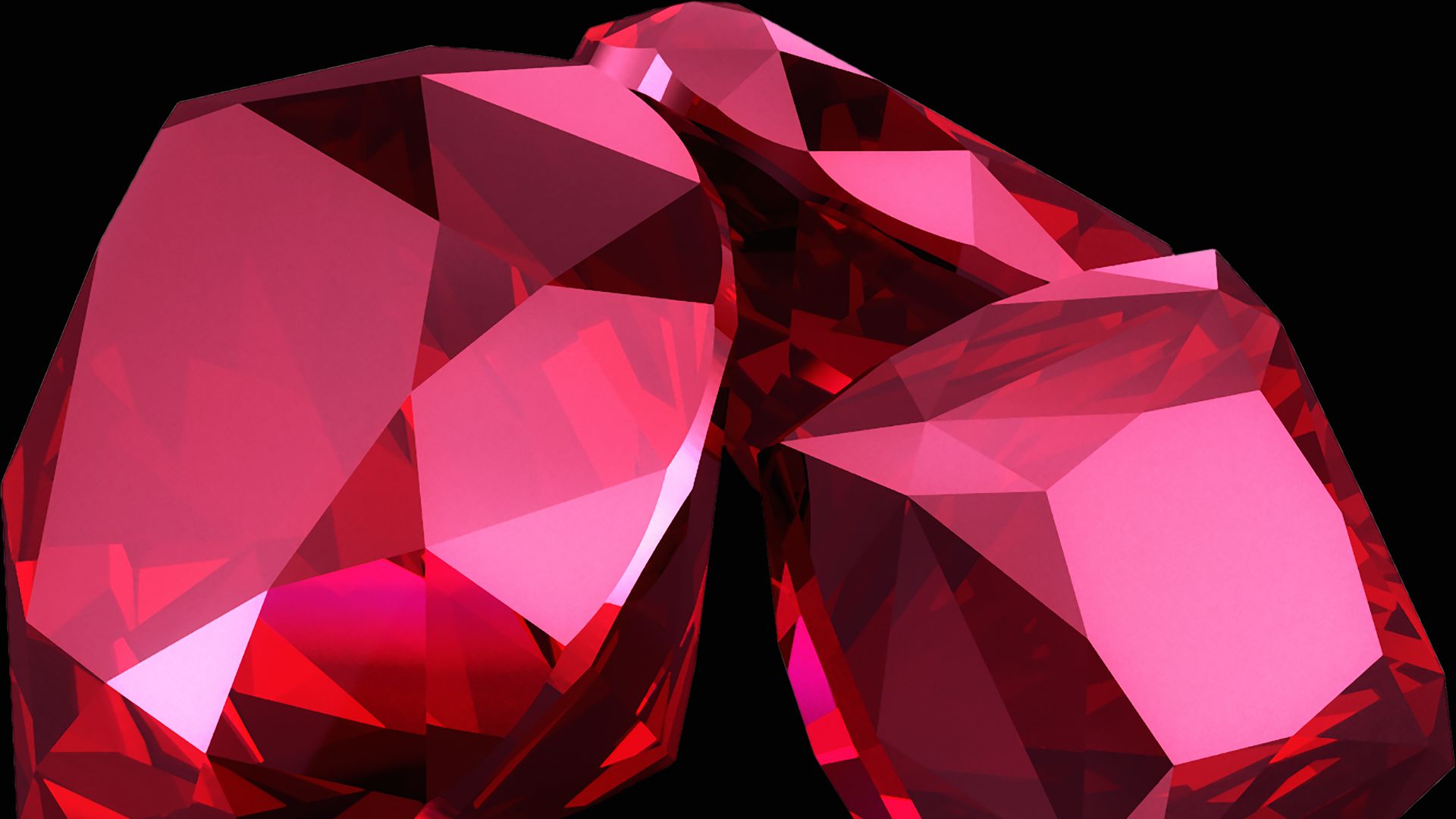
In the world of programming, data structures serve as the building blocks for efficient algorithmic solutions. Stacks and queues, often underestimated heroes in this landscape, offer distinctive methods for managing data. This section will provide a thorough exploration of implementing and utilizing stacks and queues in Ruby, shedding light on their practical applications.
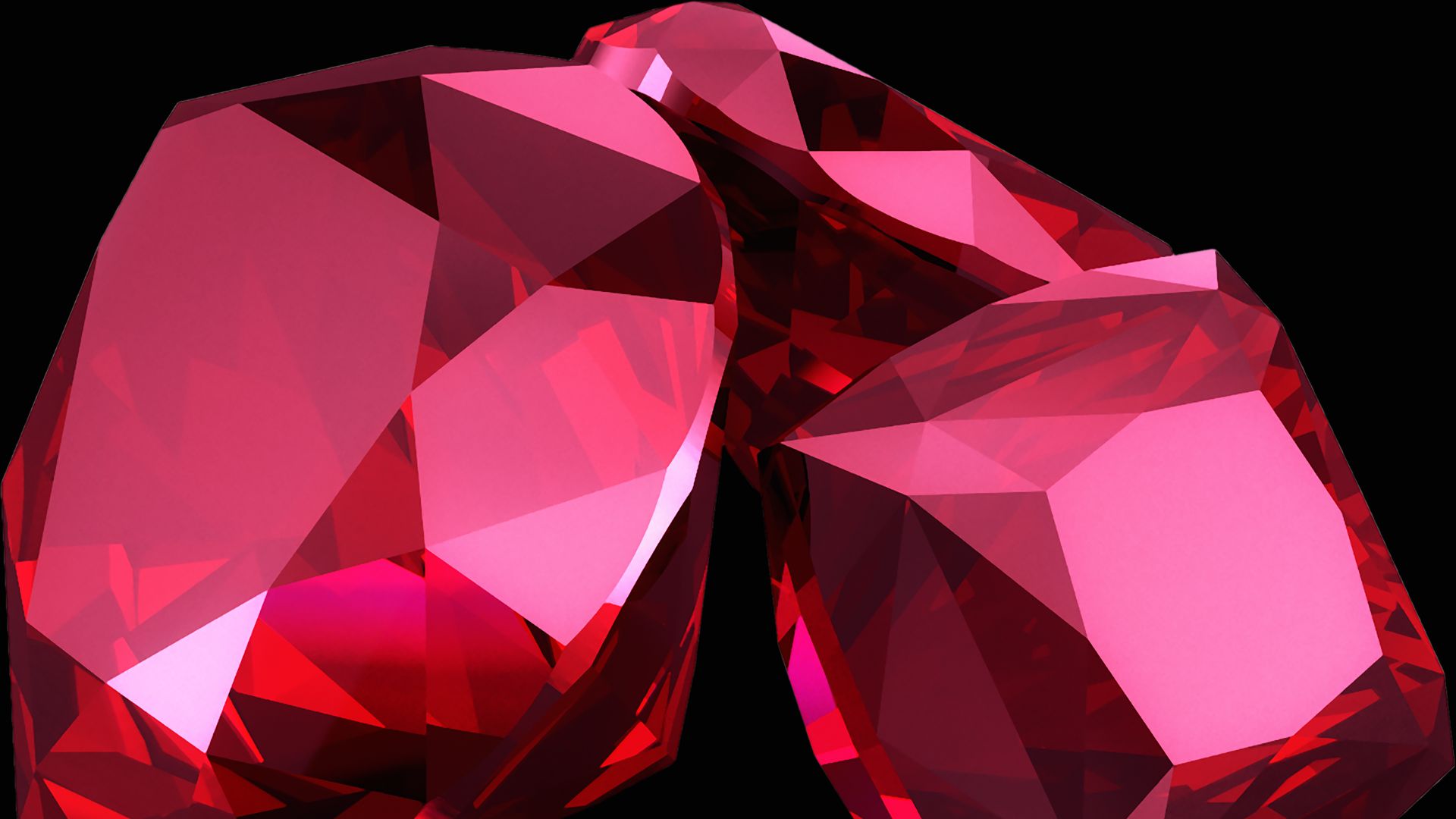
Deeper Dive into Stacks:
Understanding the Last In, First Out (LIFO) principle is crucial for grasping the essence of stacks. Picture a stack of books; the last one you place on top is the first one you pick up. In Ruby, you can implement a stack using arrays, but the real power lies in appreciating its applications.
For instance, consider the scenario of text editing where undo functionality is paramount. A stack can efficiently manage the history of changes, allowing users to backtrack through their actions seamlessly.
In-Depth Look into Queues:
Queues, governed by the First In, First Out (FIFO) concept, have their own set of applications. Imagine a queue at a ticket counter; the person who arrives first is the first to receive service. In Ruby, queues can be implemented using arrays or linked lists.
Task scheduling is a classic example where queues shine. When tasks need to be processed in the order they arrive, a queue ensures that the first task enqueued is the first to be dequeued and processed.
Strategic Decision-Making
Knowing when to deploy stacks or queues is an art in itself. Stacks prove invaluable when the order of the last few elements is critical, such as in parsing expressions or tracking browser history. On the flip side, queues are indispensable for scenarios where tasks need to be handled in a chronological order, like in print spooling or process scheduling.
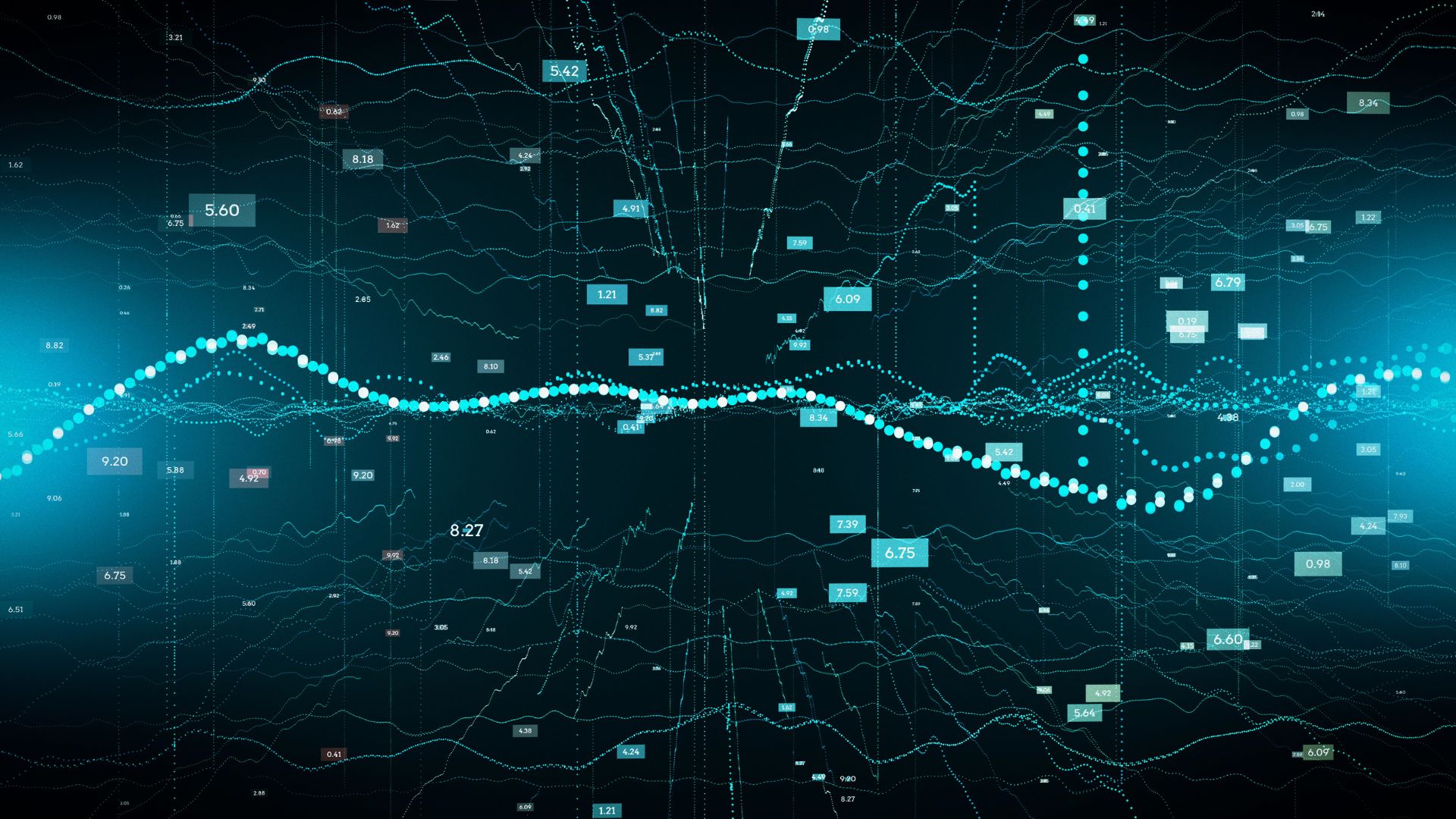