How To Implement And Use Linked Lists In Python: A Tutorial On Data Structures And Algorithms
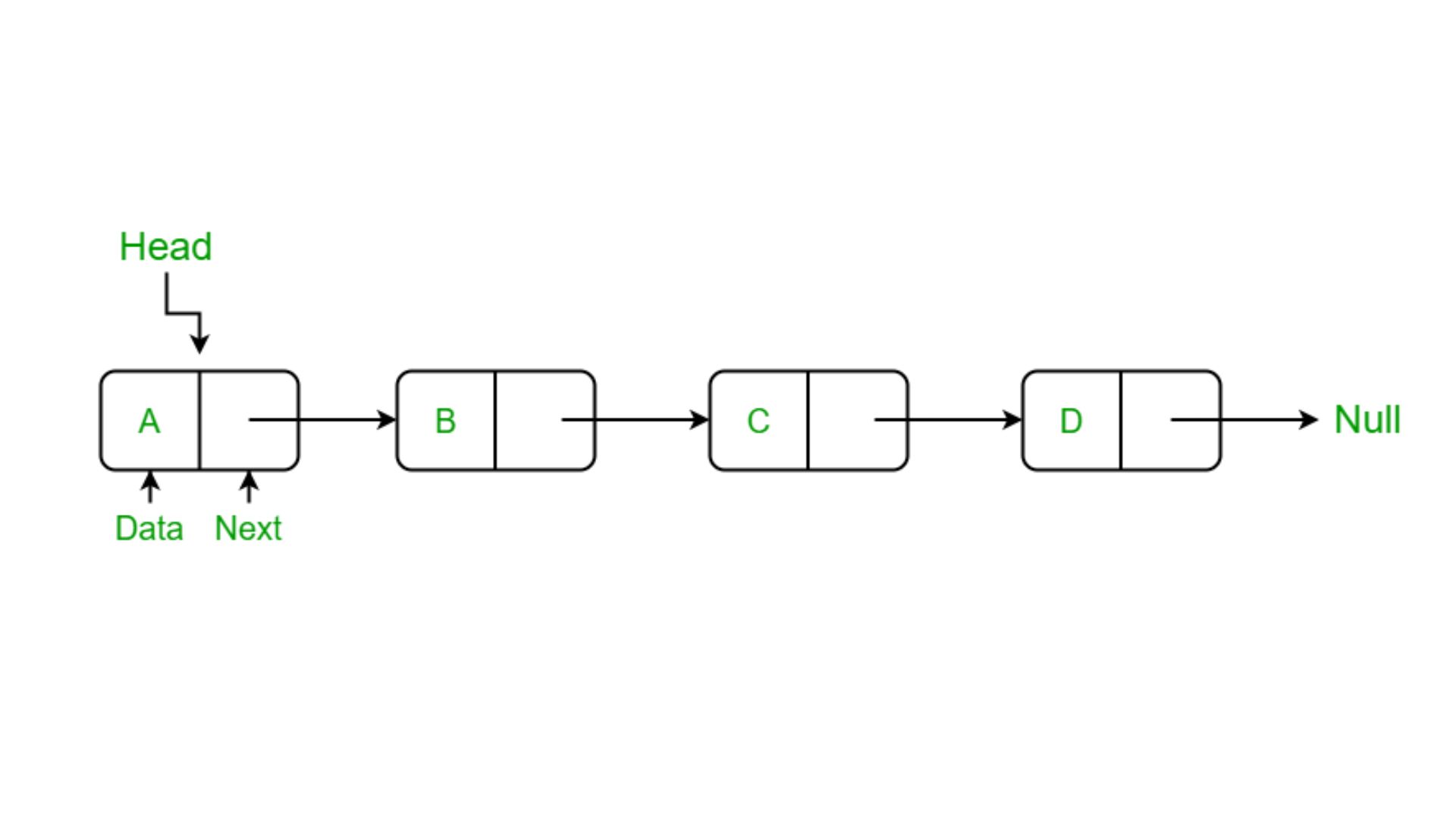
Linked lists are fundamental data structures widely used in computer science and programming. In this tutorial, we'll explore the concept of linked lists, their implementation in Python, and how they play a crucial role in building efficient data structures and algorithms.

Understanding Linked Lists
A linked list is a linear data structure where elements are stored in nodes, and each node points to the next node in the sequence. Unlike arrays, linked lists provide dynamic memory allocation, making them flexible for handling data of varying sizes.
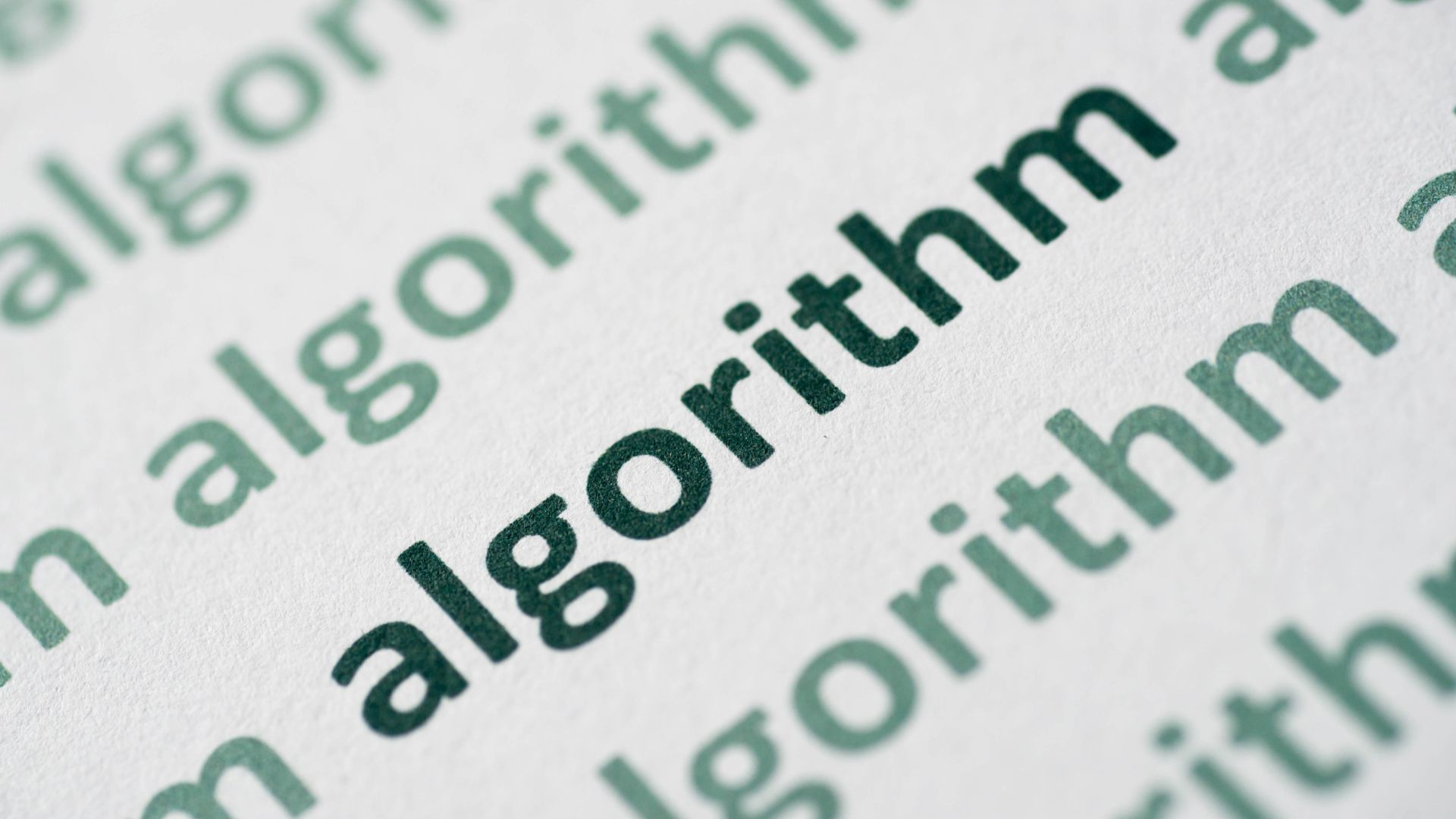
Step 1: Node Definition
In Python, a linked list is implemented using nodes. Each node contains two components: data and a reference to the next node in the sequence.
class Node:
def __init__(self, data=None):
self.data = data
self.next_node = None
Step 2: Linked List Implementation
The linked list class manages the nodes and provides methods for insertion, deletion, and traversal.
class LinkedList:
def __init__(self):
self.head = None
def insert(self, data):
new_node = Node(data)
new_node.next_node = self.head
self.head = new_node
def display(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next_node
print("None")
Step 3: Using Linked Lists in Algorithms
Linked lists are crucial in various algorithms, such as searching, sorting, and graph traversal. Their dynamic nature allows for efficient memory utilization and easy manipulation.
Step 4: Practical Example - Reversing a Linked List
def reverse_linked_list(linked_list):
current = linked_list.head
prev = None
while current:
next_node = current.next_node
current.next_node = prev
prev = current
current = next_node
linked_list.head = prev
Step 5: Benefits and Considerations
Linked lists offer advantages like constant-time insertions and deletions, but they have trade-offs, such as slower access times compared to arrays. Understanding these trade-offs is crucial when choosing the right data structure for a specific problem.
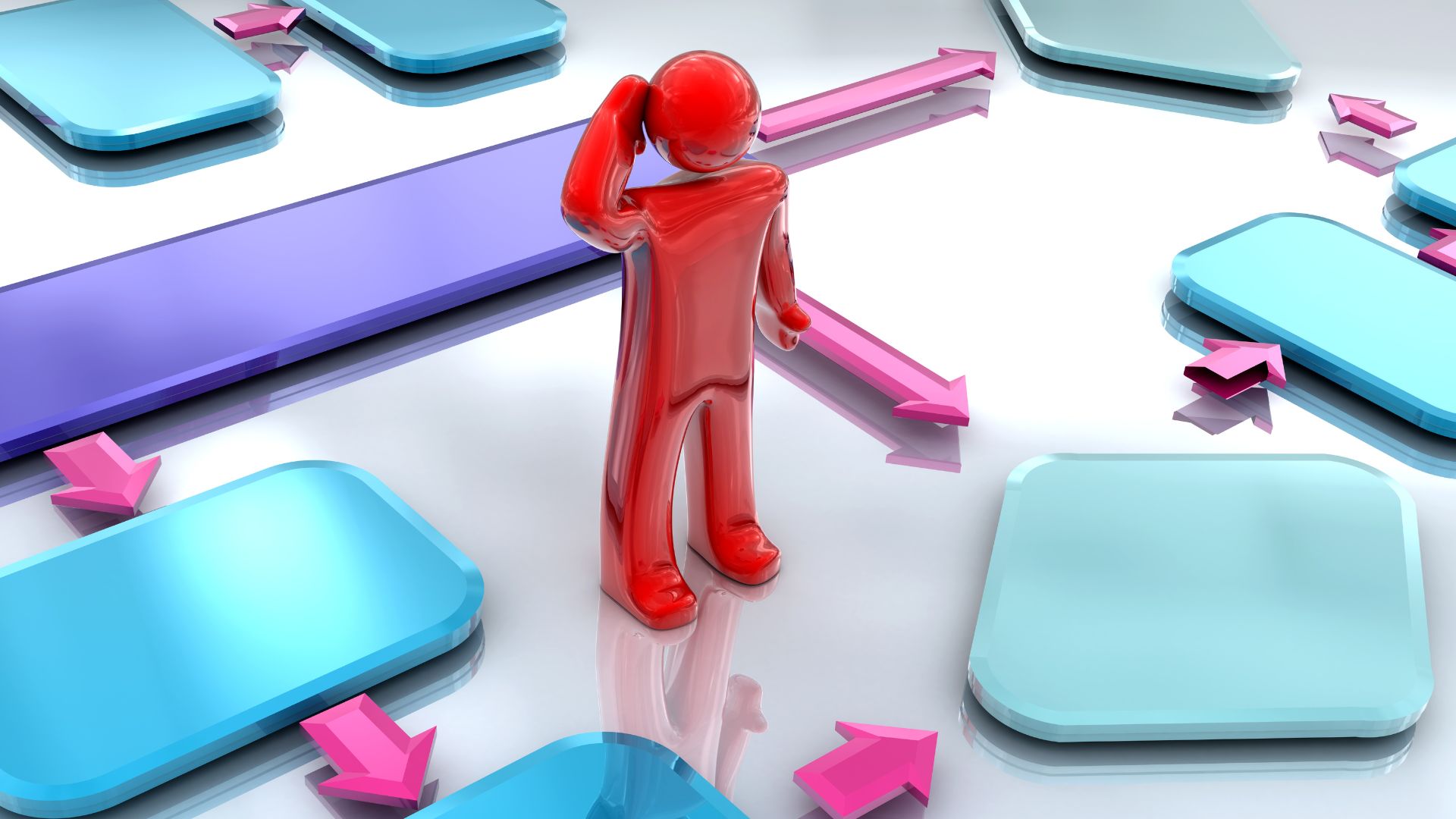
Mastering linked lists in Python opens doors to a deeper understanding of data structures and algorithms, empowering you to make informed decisions in solving diverse programming challenges.