How To Implement And Use Divide And Conquer Algorithms In Kotlin
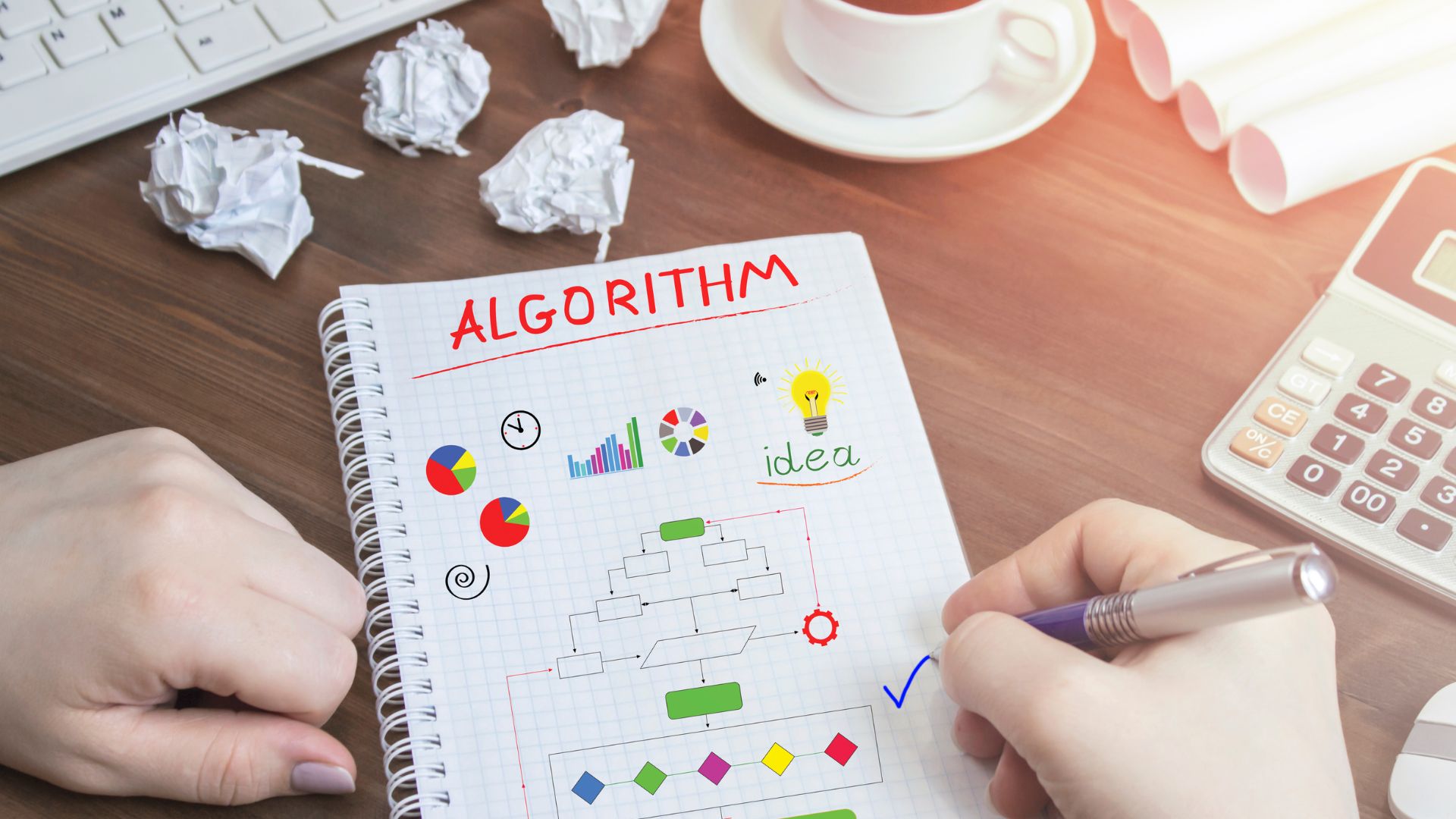
Divide and Conquer algorithms are powerful problem-solving techniques that break down complex problems into simpler sub-problems, solve them individually, and then combine their solutions to solve the original problem. Implementing and using these algorithms in Kotlin, a modern and concise programming language for the JVM, can significantly enhance your code efficiency. Hereβs a step-by-step guide on how to leverage Divide and Conquer in Kotlin.
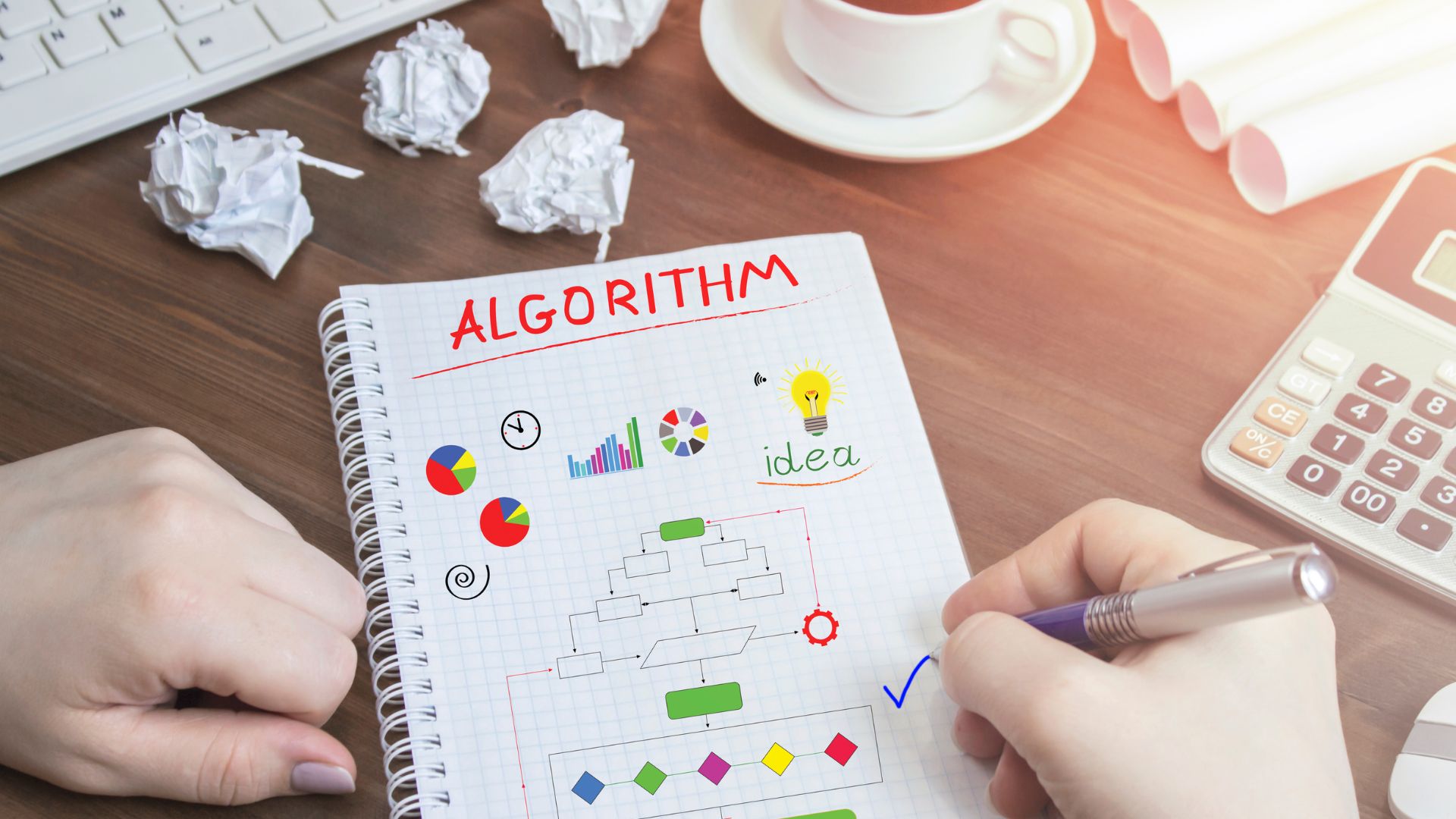
Understanding the Divide and Conquer Paradigm
Divide and Conquer involves breaking down a problem into smaller, more manageable parts. These parts are solved independently, and their solutions are then combined to solve the overall problem. This approach is particularly useful for problems that exhibit overlapping sub-problems.
Choose an Appropriate Problem
Identify a problem that can be effectively solved using Divide and Conquer. Common examples include sorting algorithms (e.g., merge sort, quicksort), searching algorithms (e.g., binary search), and certain optimization problems.
Divide Phase
Implement the divide phase by breaking the problem into smaller sub-problems. This step often involves recursion, where the original problem is divided into two or more instances of the same problem.
Conquer Phase
Write the code to solve the base cases of the problem. These are the simplest instances that can be solved directly without further division.
Combine Phase
Combine the solutions of the sub-problems to derive the solution for the original problem. This phase is critical for the efficiency and correctness of the algorithm.
Implementing in Kotlin
Kotlin's concise syntax and support for functional programming make it well-suited for implementing Divide and Conquer algorithms. Leverage Kotlin's features like tail recursion to optimize the recursive aspects of the algorithm.
Testing and Optimization
Thoroughly test your implementation with various inputs to ensure correctness. Additionally, consider optimizing the algorithm for performance, taking advantage of Kotlin's features like inline functions and immutability.
Applying Divide and Conquer in Real-World Projects
Explore real-world applications of Divide and Conquer in Kotlin. Whether it's sorting large datasets or optimizing search algorithms, the principles of Divide and Conquer can be applied to enhance the efficiency of your code.
Community and Resources
Engage with the Kotlin community to share your experiences and learn from others. There are numerous online resources, tutorials, and forums dedicated to Kotlin programming and algorithm design.
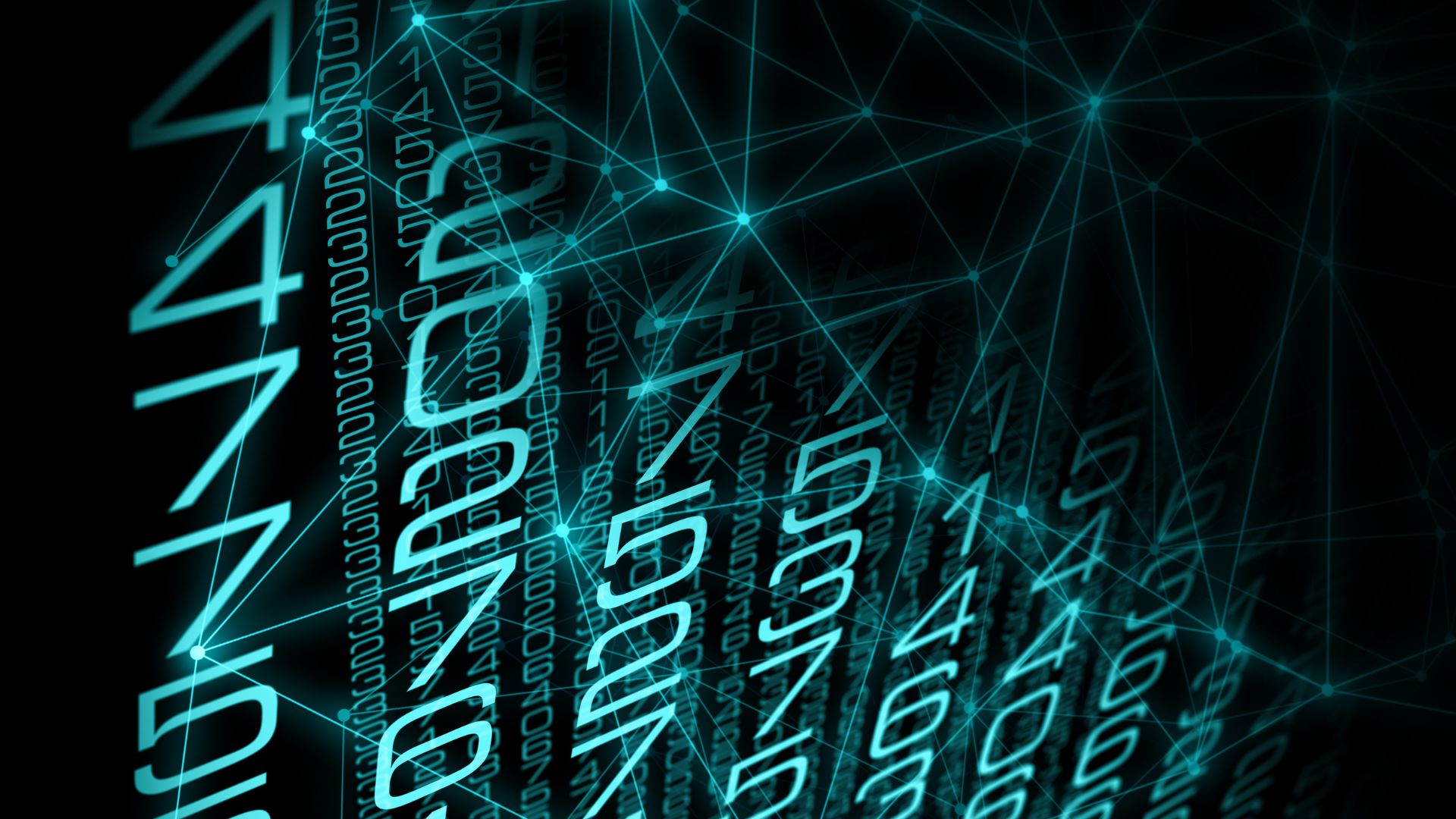
Mastering Divide and Conquer algorithms in Kotlin involves understanding the paradigm, choosing appropriate problems, and implementing the divide, conquer, and combine phases effectively. By following these steps, you can harness the power of this algorithmic technique to write efficient and scalable Kotlin code.