Basic Concepts on Data Structures and Algorithms
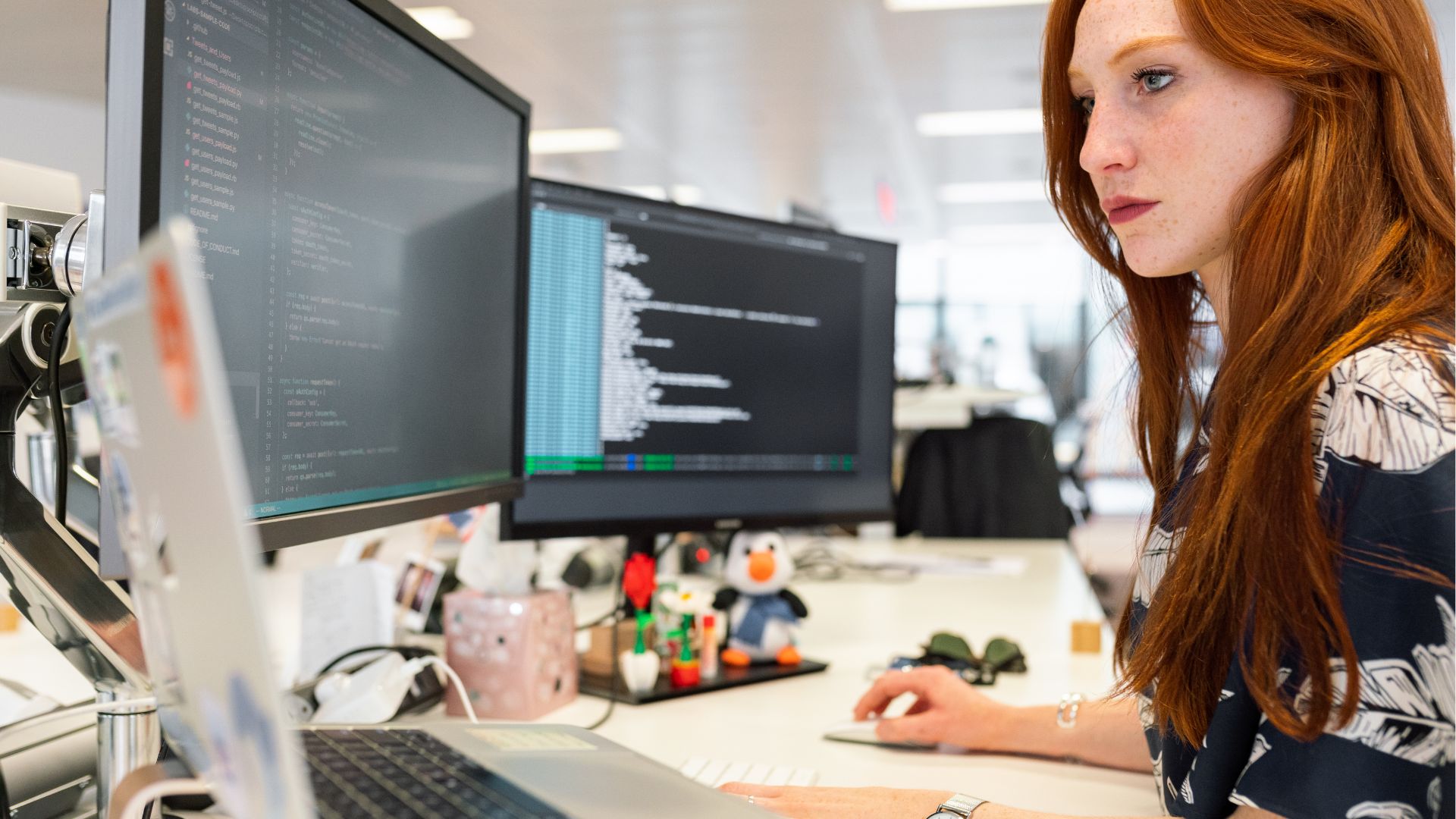
Data structures and algorithms are fundamental building blocks in computer science, providing the tools necessary for efficient problem-solving and data manipulation. Let's explore the basic concepts of data structures and algorithms and their significance in the world of programming.
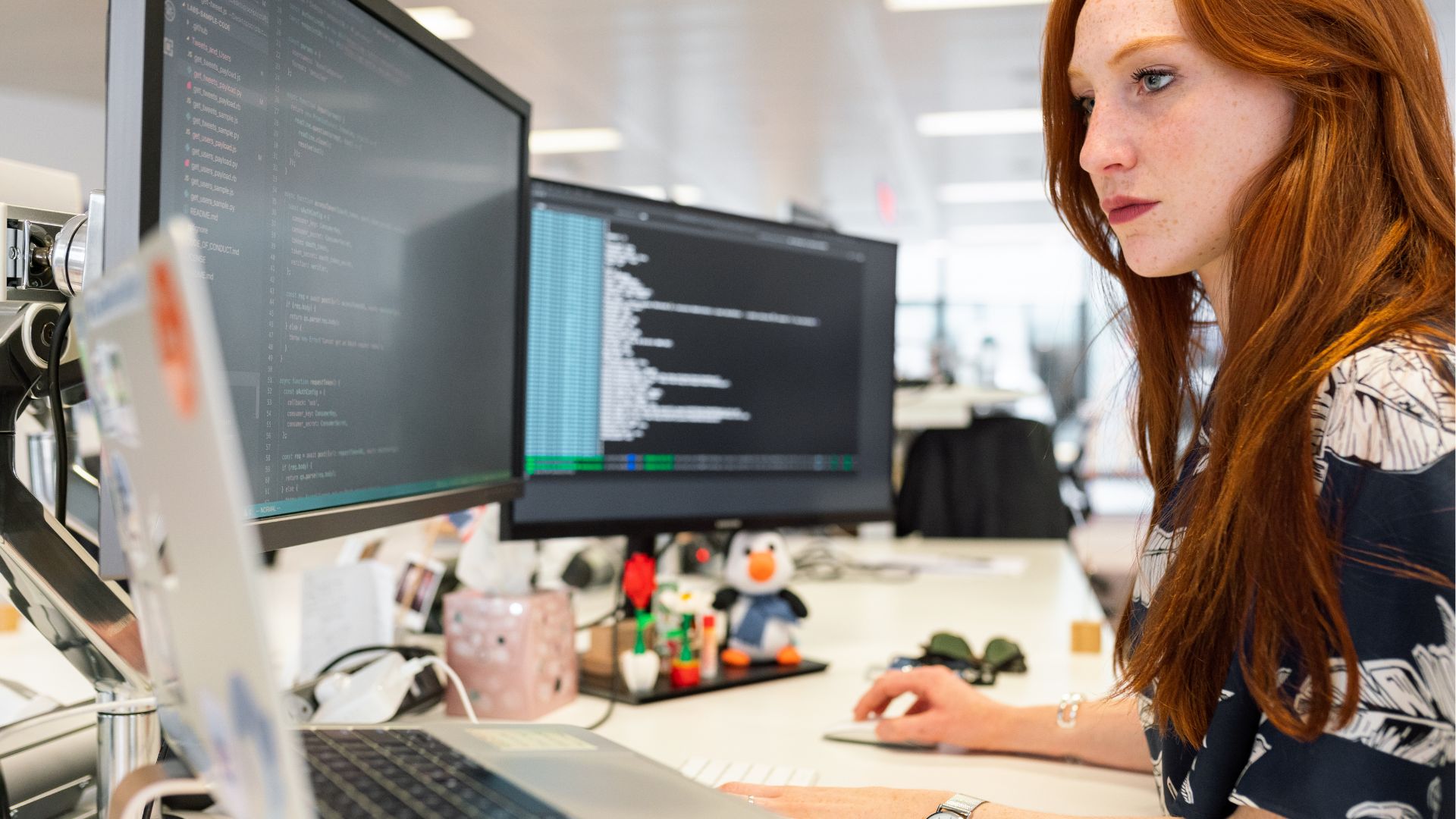
Data Structures
Data structures are organizational formats for storing and manipulating data. Common data structures include arrays, linked lists, stacks, queues, trees, and graphs. Each structure has its own strengths and weaknesses, making them suitable for specific tasks.
Algorithms
Algorithms are step-by-step procedures or formulas for solving problems. They provide a clear set of instructions to perform a specific task or solve a particular problem. Algorithms are closely tied to data structures, as the choice of data structure often influences the efficiency of the algorithm.
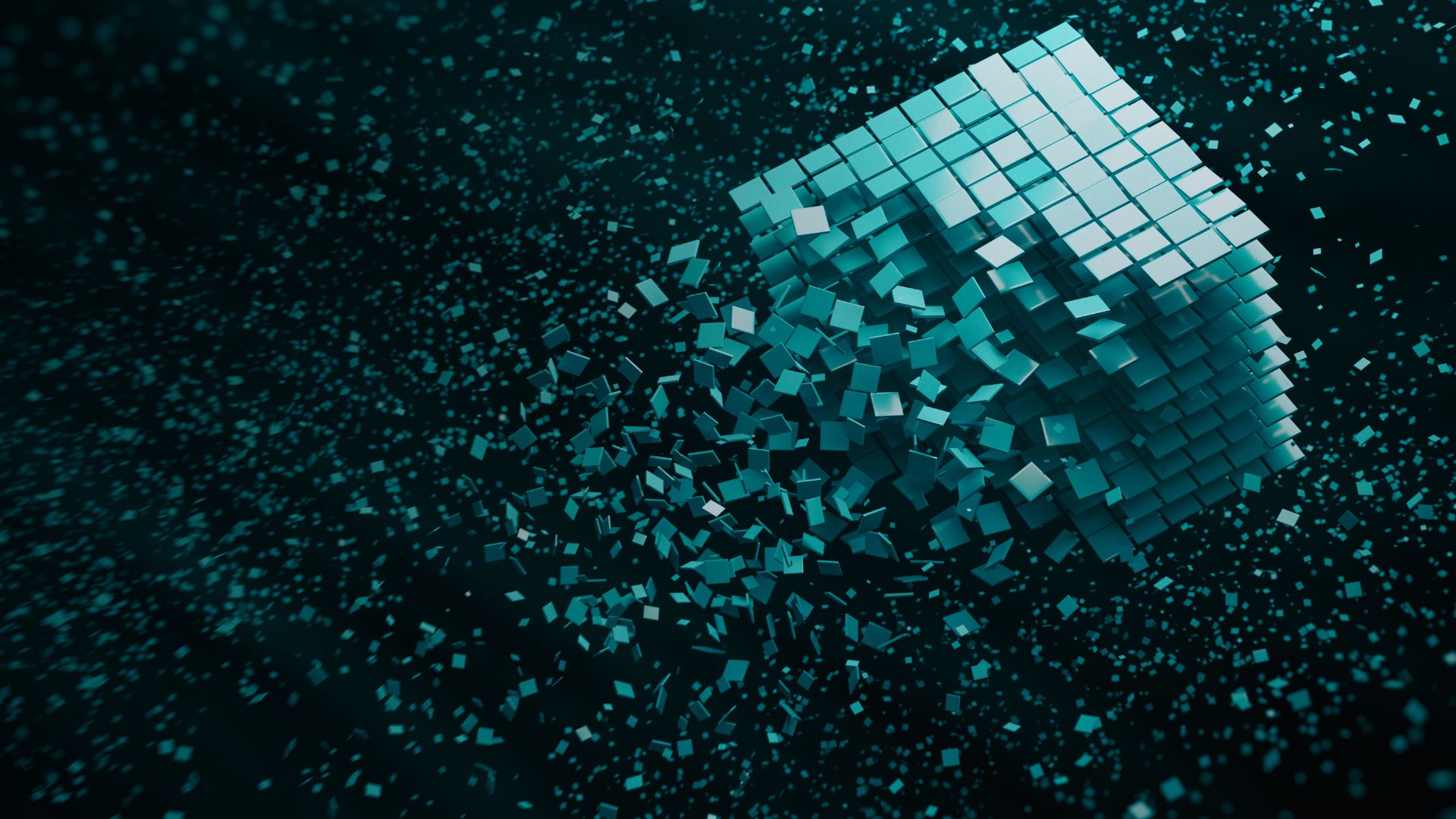
Key Concepts
- Arrays: Ordered collections of elements, accessible by index.
- Linked Lists: Elements linked together, allowing dynamic memory allocation.
- Stacks and Queues: Data structures with specific rules for adding and removing elements.
- Trees: Hierarchical structures with a root node and branches.
- Graphs: Networks of nodes and edges representing relationships.
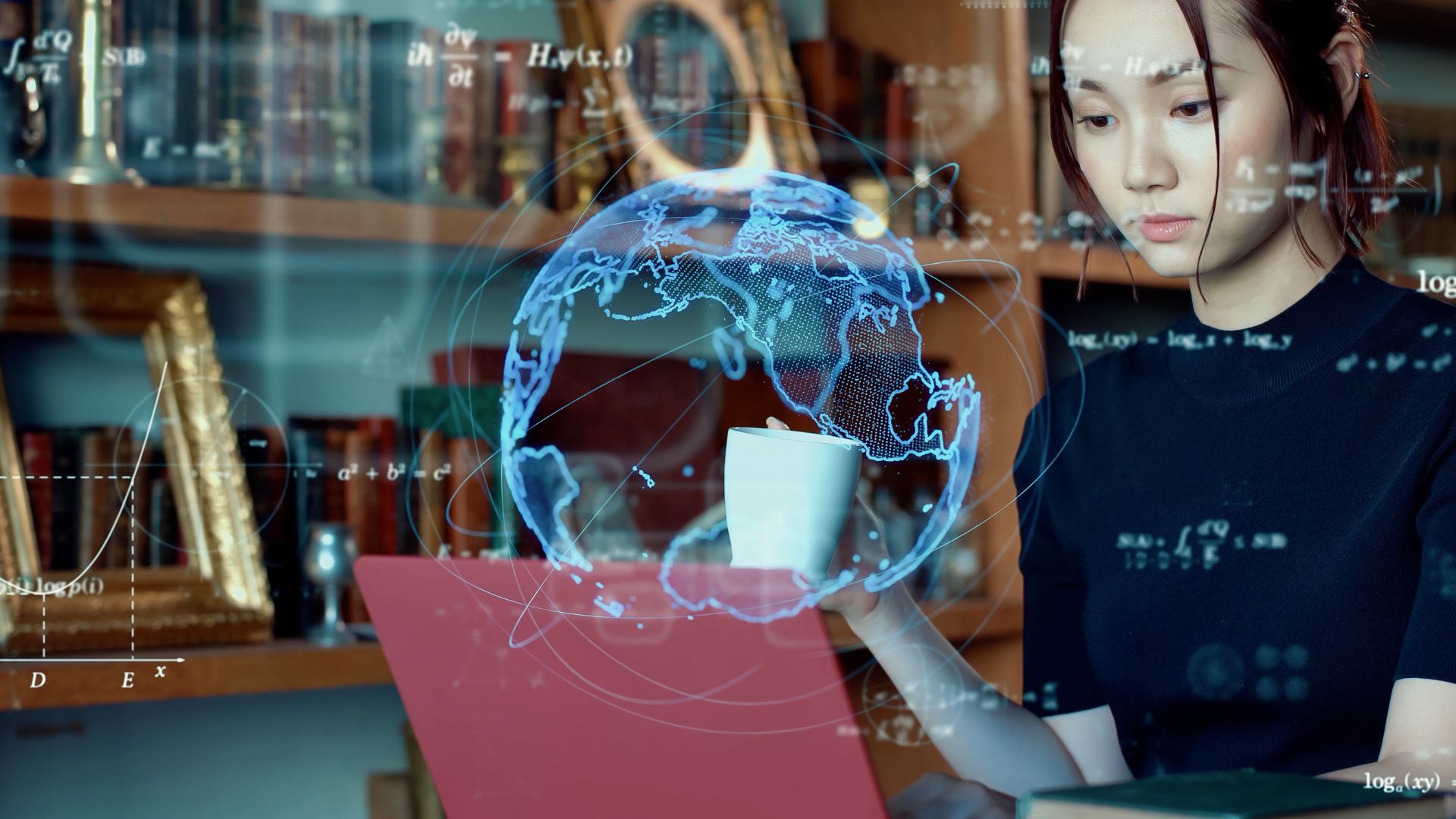
Significance in Programming
- Optimization: Efficient algorithms and data structures optimize the use of resources such as time and memory.
- Scalability: Well-designed structures and algorithms contribute to scalable and maintainable software.
- Problem Solving: The ability to choose the right data structure and algorithm is crucial for solving complex problems.
Understanding data structures and algorithms is essential for any programmer or developer. They provide the foundation for writing efficient and effective code, enabling the creation of robust software solutions that can handle diverse tasks and scenarios. Aspiring developers should focus on mastering these core concepts to excel in the ever-evolving field of computer science.